Full stack developer career path and required skills offer a dynamic and rewarding journey. Want to build entire websites and applications from front-end user interfaces to back-end databases? This path requires mastering a diverse skillset, blending creativity with technical prowess. We’ll explore the essential technologies, crucial skills, and career progression involved in becoming a successful full-stack developer.
This guide provides a comprehensive overview of the full-stack developer journey, covering everything from foundational front-end technologies like HTML, CSS, and JavaScript to back-end languages such as Python, Java, or Node.js. We’ll delve into database management, DevOps practices, version control with Git, and the essential soft skills needed for thriving in a collaborative development environment. Get ready to navigate the exciting world of full-stack development!
Introduction to the Full Stack Developer Career Path
The full-stack developer role has evolved significantly since its inception. Initially, it often described developers with a broad understanding of both front-end and back-end technologies, capable of handling most aspects of a project. However, as technologies have become more complex and specialized, the definition has broadened, and the skillset required has become significantly more demanding. Today, a full-stack developer is expected to possess a deep understanding of a wider range of technologies and be proficient in multiple programming languages and frameworks.The demand for full-stack developers continues to grow, fueled by the ever-increasing reliance on web and mobile applications.
This has led to a clear career progression path, offering numerous opportunities for growth and specialization.
Typical Career Progression for a Full-Stack Developer, Full stack developer career path and required skills
A typical career progression often begins with a junior full-stack developer role, focusing on gaining experience and building a strong foundation in core technologies. This stage involves working on smaller projects under the supervision of senior developers, learning to implement designs, write clean code, and collaborate effectively within a team. With experience, a developer progresses to a mid-level role, where they take on more responsibility, lead smaller projects, and contribute to architectural decisions.
Senior full-stack developers typically lead larger projects, mentor junior team members, and play a crucial role in shaping the technological direction of a company. Many eventually move into architect or team lead roles, managing projects and teams, and overseeing the entire software development lifecycle.
Specializations Within Full-Stack Development
While the term “full-stack” implies a broad skillset, specialization within this field is becoming increasingly common. Some developers might specialize in specific technologies, such as React, Node.js, or Python/Django. Others may focus on specific areas of development, such as mobile app development (using frameworks like React Native or Flutter), data science integration, or DevOps. For example, a developer might specialize in building high-performance backend systems using Java and Spring Boot, while another might focus on creating user-friendly and visually appealing front-ends using React and Redux.
The possibilities are vast and often driven by individual interests and market demand. The specialization allows for deeper expertise in a particular area, leading to higher earning potential and increased job satisfaction.
Essential Front-End Skills
Building amazing websites and web applications requires a solid understanding of front-end development. This involves crafting the user interface (UI) and user experience (UX) – everything the user directly interacts with. Let’s dive into the core technologies and concepts.
Core Front-End Technologies
HTML, CSS, and JavaScript form the holy trinity of front-end development. They work together seamlessly to create dynamic and visually appealing websites. HTML provides the structure, CSS the styling, and JavaScript the interactivity.
HTML (HyperText Markup Language) is the foundation. It uses tags to define elements like headings, paragraphs, images, and links, creating the skeletal structure of a webpage. Think of it as the blueprint of your website.
CSS (Cascading Style Sheets) handles the visual presentation. It dictates colors, fonts, layout, and responsiveness, transforming the basic HTML structure into a visually engaging experience. It’s the website’s aesthetic coat of paint.
JavaScript adds interactivity and dynamism. It allows for things like animations, form validation, dynamic content updates, and much more. It brings the website to life, making it responsive and engaging for the user.
Responsive Web Design Principles
Responsive design ensures your website adapts seamlessly to different screen sizes – from desktops to tablets to smartphones. This is crucial for providing a consistent and optimal user experience across all devices. Key principles include using flexible layouts (using percentages instead of fixed pixel values), flexible images (scaling images appropriately), and media queries (applying different CSS styles based on screen size).
A website that isn’t responsive will likely frustrate users and negatively impact your reach. For example, a website designed only for desktops will be difficult to navigate on a mobile phone.
Comparison of JavaScript Frameworks
Several JavaScript frameworks simplify and streamline front-end development. React, Angular, and Vue.js are three of the most popular, each with its strengths and weaknesses.
Feature | React | Angular | Vue.js |
---|---|---|---|
Learning Curve | Relatively easy, component-based approach | Steeper learning curve, requires understanding of TypeScript and Angular concepts | Gentle learning curve, easy to integrate into existing projects |
Data Binding | One-way data binding, with some two-way binding libraries | Two-way data binding | Two-way data binding |
Community Support | Large and active community | Large and active community | Growing rapidly, strong community support |
Scalability | Highly scalable, suitable for large applications | Highly scalable, designed for large-scale enterprise applications | Highly scalable, suitable for both small and large applications |
Sample User Interface Design
Let’s design a simple login form using HTML, CSS, and JavaScript.
HTML (index.html):
<!DOCTYPE html>
<html>
<head>
<title>Login Form</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="container">
<h1>Login</h1>
<form id="loginForm">
<input type="text" id="username" placeholder="Username">
<input type="password" id="password" placeholder="Password">
<button type="submit">Login</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
CSS (style.css):
.container
width: 300px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
JavaScript (script.js):
Want a full-stack developer career? You’ll need skills in front-end (HTML, CSS, JavaScript), back-end (like Python or Node.js), and databases. Getting started can feel overwhelming, but check out top-rated IT courses for beginners with career guidance to find a structured learning path. These courses often cover the foundational skills you need to build a solid base before tackling more advanced full-stack concepts.
Mastering these fundamentals is key to a successful career.
document.getElementById('loginForm').addEventListener('submit', function(event)
event.preventDefault();
// Add your login logic here
);
This simple example shows a login form with a username and password field. The CSS provides basic styling, and the JavaScript prevents the default form submission behavior, allowing for custom login logic to be added.
Essential Back-End Skills
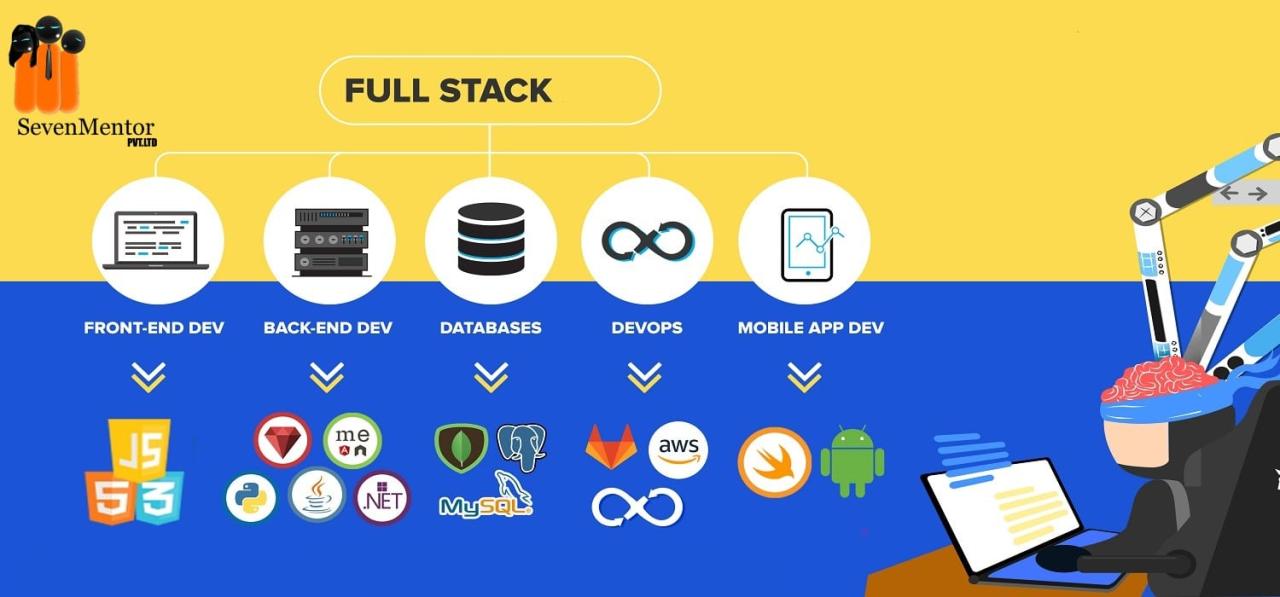
Building the back-end of a web application involves a lot more than just writing code. It’s about creating the robust, secure, and scalable foundation that powers the user experience. This section covers the core skills needed to excel in back-end development.
A solid grasp of back-end technologies is crucial for any full-stack developer. This includes understanding programming languages, databases, API architectures, and security best practices. Mastering these elements allows you to build efficient, reliable, and secure applications.
Popular Back-End Programming Languages
Back-end development relies heavily on server-side programming languages. Choosing the right language often depends on the project’s specific needs and the developer’s expertise. However, several languages consistently rank among the most popular and versatile options.
Python, known for its readability and extensive libraries, is a favorite for its ease of use and versatility. It excels in machine learning, data science, and web development, often used with frameworks like Django and Flask. Java, a robust and mature language, is renowned for its scalability and reliability, making it ideal for large-scale enterprise applications. Its ecosystem includes powerful frameworks like Spring.
Node.js, built on JavaScript, allows developers to use the same language for both front-end and back-end development, improving efficiency and streamlining workflows. It’s popular for real-time applications and APIs.
The Role of Databases in Back-End Development
Databases are the heart of most back-end systems, responsible for storing and managing application data. Understanding the different types of databases and their strengths is vital for building efficient and scalable applications.
SQL databases, like MySQL, PostgreSQL, and SQL Server, are relational databases that organize data into tables with rows and columns. They are well-suited for structured data and offer strong data integrity features. NoSQL databases, such as MongoDB and Cassandra, are non-relational databases that offer more flexibility in data modeling. They are often preferred for unstructured or semi-structured data and applications requiring high scalability and availability.
The choice between SQL and NoSQL depends on the specific needs of the application; some applications even utilize both types.
API Architectures: REST and GraphQL
Application Programming Interfaces (APIs) are the communication channels between different parts of an application or between different applications. Understanding different API architectures is essential for designing efficient and scalable systems.
REST (Representational State Transfer) is a widely used architectural style for building web APIs. It relies on standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources. GraphQL, a query language for APIs, provides a more efficient way to fetch data by allowing clients to request only the data they need. While REST is well-established and widely supported, GraphQL’s efficiency in data fetching makes it a strong contender for modern applications.
Server-Side Security
Security is paramount in back-end development. Protecting user data and preventing unauthorized access is crucial for maintaining the integrity and trustworthiness of an application.
Implementing robust security measures, including input validation, authentication, authorization, and encryption, is essential. Regular security audits and penetration testing can identify vulnerabilities and help prevent attacks. Following secure coding practices and staying up-to-date on the latest security threats are also vital aspects of back-end security.
Best Practices for Back-End Code Organization and Maintainability
Well-organized and maintainable code is crucial for long-term success. Following best practices ensures that the code is easy to understand, modify, and debug.
Using version control (like Git), writing clean and well-documented code, following consistent coding style guidelines, and employing modular design principles are key practices. Regular code reviews and testing (unit, integration, and end-to-end) help identify and fix bugs early in the development process. Employing design patterns and utilizing a well-defined architecture can greatly enhance code maintainability and scalability.
Essential DevOps Skills
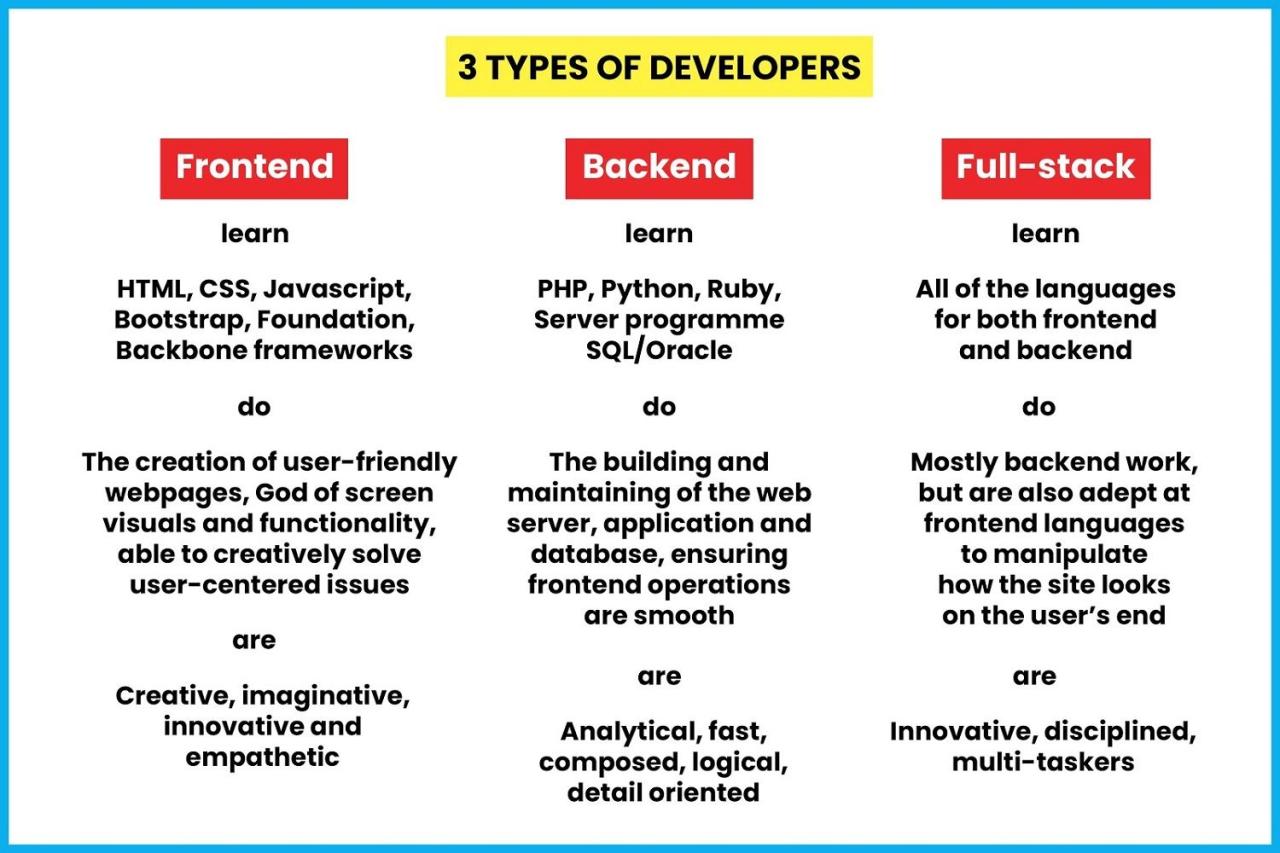
DevOps is a crucial set of practices that bridges the gap between development and operations teams. In the context of full-stack development, understanding and implementing DevOps principles is essential for building, deploying, and maintaining applications efficiently and reliably. It’s about automation, collaboration, and continuous improvement throughout the entire software lifecycle.DevOps practices streamline the development process, leading to faster releases, improved stability, and increased collaboration among team members.
This ultimately results in higher quality software delivered more quickly and effectively. A strong grasp of DevOps is increasingly important for full-stack developers aiming for senior roles and contributing to larger projects.
DevOps Tools and Practices
Understanding and using common DevOps tools and practices is vital for a full-stack developer. These tools automate tasks, improve collaboration, and ensure consistent deployments.
- Version Control (Git): Git is the industry-standard version control system. It allows developers to track changes to their code, collaborate effectively, and manage different versions of a project. Features like branching, merging, and pull requests are fundamental to collaborative development and managing code efficiently.
- Continuous Integration/Continuous Delivery (CI/CD): CI/CD is a set of practices that automates the process of building, testing, and deploying software. Continuous Integration focuses on frequently integrating code changes into a central repository, while Continuous Delivery automates the release process to various environments (development, testing, production).
Examples of DevOps Improving the Development Lifecycle
Implementing DevOps significantly enhances the software development lifecycle.
- Faster Releases: Automation reduces manual effort, allowing for more frequent and rapid releases of new features and bug fixes. Instead of weeks or months between releases, DevOps enables deployments several times a day or week.
- Improved Collaboration: DevOps fosters better communication and collaboration between development and operations teams. Shared responsibility and automated processes reduce friction and improve efficiency.
- Increased Reliability: Automated testing and monitoring help identify and resolve issues early in the development process, leading to more reliable and stable applications. Continuous monitoring and automated rollbacks minimize downtime.
Cloud Platform Comparison
Several major cloud platforms offer services supporting DevOps practices. Each has its strengths and weaknesses, and the best choice depends on specific project needs.
Feature | AWS | Azure | Google Cloud |
---|---|---|---|
Pricing | Pay-as-you-go, various pricing models | Pay-as-you-go, various pricing models | Pay-as-you-go, various pricing models |
Services | Extensive range of services for compute, storage, databases, etc. | Comprehensive suite of services mirroring AWS, with strong integration with Microsoft products. | Strong in data analytics and machine learning, with a growing range of general-purpose services. |
Market Share | Largest market share | Second largest market share | Significant market share, strong in specific areas |
CI/CD Pipeline Flowchart
A typical CI/CD pipeline involves several stages. The following describes a simplified visual representation:Imagine a flowchart. It starts with a “Code Commit” box, which feeds into a “Build” box. The “Build” box then leads to a “Test” box, which branches into two paths: one leading to a “Deploy to Staging” box and the other to a “Fail” box (if tests fail).
The “Deploy to Staging” box then leads to a “Manual Approval” box. If approved, it moves to “Deploy to Production,” and if not, it goes back to “Code Commit” for adjustments. Finally, both “Deploy to Staging” and “Deploy to Production” boxes lead to a “Monitoring” box, which constantly tracks the application’s performance and health. If issues are detected, it can trigger a rollback or further investigation.
Database Management Skills
As a full-stack developer, you’ll be interacting with databases constantly. Understanding how to choose, design, and manage databases is crucial for building robust and scalable applications. This section covers essential database management skills, focusing on the differences between relational and NoSQL databases, the importance of database design, and practical examples of SQL queries.
Relational vs. NoSQL Databases
Relational databases (RDBMS), like MySQL, PostgreSQL, and SQL Server, organize data into tables with rows and columns, enforcing relationships between them. NoSQL databases, on the other hand, offer more flexible data models, such as document, key-value, graph, or column-family stores. Examples include MongoDB, Cassandra, and Redis. The choice between them depends on the application’s specific needs. RDBMS excel in structured data scenarios requiring ACID properties (Atomicity, Consistency, Isolation, Durability), ensuring data integrity.
NoSQL databases are better suited for large volumes of unstructured or semi-structured data, prioritizing scalability and availability.
Database Normalization and Design
Database normalization is the process of organizing data to reduce redundancy and improve data integrity. It involves breaking down larger tables into smaller ones and defining relationships between them. Proper database design is crucial for performance and maintainability. A well-designed database minimizes data redundancy, improves query efficiency, and simplifies data updates. Poor database design, conversely, can lead to performance bottlenecks and data inconsistencies.
Normalization typically involves several normal forms (1NF, 2NF, 3NF, etc.), each addressing specific redundancy issues.
Common SQL Queries
SQL (Structured Query Language) is the standard language for interacting with relational databases. Here are examples of four fundamental SQL queries:
- SELECT: Retrieves data from a database. Example:
SELECT
This query selects all columns (*) from the ‘users’ table where the ‘age’ column is greater than 25.
- FROM users WHERE age > 25; - INSERT: Adds new data into a database. Example:
INSERT INTO users (name, age, email) VALUES ('John Doe', 30, '[email protected]');
This inserts a new row into the ‘users’ table with the specified values. - UPDATE: Modifies existing data in a database. Example:
UPDATE users SET age = 31 WHERE name = 'John Doe';
This updates the ‘age’ column to 31 for the user named ‘John Doe’. - DELETE: Removes data from a database. Example:
DELETE FROM users WHERE id = 1;
This deletes the row with the ID of 1 from the ‘users’ table.
Using a Database Management Tool
Database management tools provide a graphical interface for creating, managing, and interacting with databases. Popular tools include phpMyAdmin (for MySQL), pgAdmin (for PostgreSQL), and SQL Server Management Studio (for SQL Server). These tools allow users to create and modify databases, tables, and views, execute SQL queries, and manage users and permissions, simplifying database administration significantly. For example, using phpMyAdmin, one can visually create a new database, add tables with specific columns and data types, and then execute SQL queries to manipulate the data without writing raw SQL commands directly into a terminal.
Comparison of Database Types
Feature | Relational Databases (SQL) | NoSQL Databases |
---|---|---|
Data Model | Structured, tabular | Flexible (document, key-value, graph, etc.) |
Scalability | Vertical scaling is easier, horizontal scaling more complex | Horizontal scaling is generally easier |
Data Integrity | High, enforced through constraints | Lower, requires application-level enforcement |
ACID Properties | Strong support | Often weaker or absent |
Version Control and Collaboration
Version control is absolutely crucial for any software development project, especially when working collaboratively. It’s like having a detailed history of your code, allowing you to track changes, revert to previous versions, and work efficiently with others without stepping on each other’s toes. Think of it as a safety net and a powerful tool for managing your project’s evolution.
Git is the industry-standard version control system, and understanding its core principles is essential for any full-stack developer.Git allows developers to manage changes to their codebase over time. This includes tracking modifications, collaborating effectively with team members, and resolving conflicts that inevitably arise during concurrent development. The ability to roll back to previous versions is invaluable in debugging and maintaining software stability.
So you’re thinking about a full stack developer career path? It’s a challenging but rewarding journey! You’ll need a solid grasp of front-end and back-end technologies, plus database management skills. To really understand what this entails, check out this resource on becoming a full stack developer. Learning this diverse skillset will open many doors, ensuring a fulfilling and adaptable career path for years to come.
Mastering these skills is key to succeeding as a full stack developer.
Git Commands
Understanding a few core Git commands is essential for effective version control. These commands are the building blocks of managing your code’s history and collaborating with others.
git commit
: This saves your changes locally. Think of it as taking a snapshot of your work at a specific point in time, along with a descriptive message explaining the changes made. This message is incredibly important for future reference and understanding the evolution of the code.git push
: This uploads your local commits to a remote repository (like GitHub, GitLab, or Bitbucket). This makes your changes available to others working on the project.git pull
: This downloads changes from a remote repository to your local machine. This ensures you have the latest version of the codebase before making any further changes.git branch
: This creates and manages branches. Branches allow developers to work on new features or bug fixes independently without affecting the main codebase (often called the “main” or “master” branch). This is crucial for parallel development and avoiding conflicts.git merge
: This combines changes from one branch into another. This is how you integrate your work on a feature branch back into the main branch once it’s complete and tested.
Collaborative Coding Best Practices with Git
Effective collaboration with Git requires adherence to some best practices. These practices streamline workflows, prevent conflicts, and ensure a smooth development process.
- Frequent Commits with Clear Messages: Commit often, with concise but descriptive messages explaining the purpose of each commit. This makes it easy to track changes and understand the code’s evolution.
- Use Branches Effectively: Create branches for new features or bug fixes. This isolates changes and allows parallel development without disrupting the main codebase.
- Pull Regularly: Regularly pull changes from the remote repository to ensure you’re working with the latest version of the code.
- Resolve Conflicts Promptly: When conflicts arise (due to simultaneous changes to the same lines of code), address them immediately and commit the resolution.
- Code Reviews: Utilize code review features (often integrated into platforms like GitHub) to have others review your code before merging it into the main branch. This improves code quality and catches potential issues early.
Managing Code Changes and Resolving Conflicts
Imagine two developers, Alice and Bob, working on the same file. Alice adds a new function, and Bob modifies an existing one. If they both push their changes without pulling the latest version, a conflict arises. Git will mark the conflicting sections, and they need to manually resolve them by deciding which changes to keep or how to combine them.
This is where a clear commit history and good communication become essential. Git will highlight the conflicting sections in the file, allowing Alice and Bob to carefully review and choose the best approach.
Git Learning Resources
There are many excellent resources available to help you learn Git and improve your version control skills.
- GitHub Learning Lab: Offers interactive courses and tutorials on Git and GitHub.
- Atlassian Git Tutorials: Provides comprehensive guides and documentation on Git and Bitbucket.
- Git documentation: The official Git documentation is a great resource for in-depth information.
- Online Courses (Coursera, Udemy, etc.): Many platforms offer structured courses on Git and version control.
Problem-Solving and Debugging Skills
Debugging is a fundamental skill for any full-stack developer. It’s not just about fixing errors; it’s about understanding how your code works, identifying the root cause of problems, and implementing effective solutions. Mastering debugging techniques significantly improves your efficiency and reduces development time.Debugging involves systematically identifying and resolving errors in your code. This process requires patience, attention to detail, and a methodical approach.
While frustrating at times, effective debugging is crucial for building reliable and high-quality applications. The ability to quickly and efficiently debug code is a highly valued skill in the software development industry.
Front-End and Back-End Debugging Techniques
Front-end and back-end debugging differ slightly in their approach due to the distinct nature of the code and the tools available. Front-end debugging often involves using browser developer tools to inspect the HTML, CSS, and JavaScript code, while back-end debugging usually relies on logging, debugging tools within the development environment (like IDE debuggers), and examining server logs. Both, however, share the core principles of systematic investigation and error isolation.
The Importance of Debugging Tools and Browser Developer Tools
Debugging tools are invaluable assets for developers. Browser developer tools, specifically, provide a comprehensive suite of features for inspecting the front-end code, including setting breakpoints, stepping through code execution, examining variables, and profiling performance. Similarly, IDE debuggers for back-end development allow developers to set breakpoints, step through code, inspect variables, and examine the call stack, greatly aiding in pinpointing the source of errors.
Using these tools efficiently significantly reduces the time spent troubleshooting. Ignoring these tools is like trying to fix a car engine blindfolded.
Common Programming Errors and Their Solutions
Many common programming errors stem from simple mistakes, such as typos, incorrect syntax, or logical errors. For example, a common JavaScript error is a `TypeError` which often occurs when trying to perform an operation on a variable that’s not of the expected type (e.g., adding a number to a string). In back-end development, using an incorrect database query or failing to handle exceptions properly can lead to application crashes or unexpected behavior.
Careful code review, comprehensive testing, and the use of linters can help prevent many of these errors. Understanding the specific error messages provided by the compiler or interpreter is crucial in identifying and rectifying the problem.
Strategies for Troubleshooting Complex Software Issues
Troubleshooting complex issues requires a more systematic approach. Start by reproducing the problem consistently. Then, break down the problem into smaller, more manageable parts. Use logging statements to track the flow of execution and the values of variables. Isolate the problem by commenting out sections of code to identify the problematic area.
Consult documentation, online resources, and colleagues for assistance when needed. Remember to test your solutions thoroughly after implementing them to ensure that you have not introduced new problems.
Debugging a Simple Application: A Step-by-Step Guide
Let’s imagine a simple web application that displays a user’s name. If the name doesn’t display correctly, here’s how to debug:
1. Reproduce the error
Try different inputs to see if the problem is consistent.
2. Inspect the HTML
Use your browser’s developer tools to check if the name is correctly rendered in the HTML. Is the element present? Is the correct value assigned?
3. Examine the JavaScript (if applicable)
If the name is fetched dynamically via JavaScript, set breakpoints in your debugger to step through the code, inspect the value of the name variable, and check for any errors during the fetching or rendering process.
4. Check the server-side code (if applicable)
If the name is retrieved from a database or an API, verify that the server-side code correctly retrieves and returns the data. Examine the server logs for any errors.
5. Test thoroughly
After making changes, test the application extensively to ensure that the error is resolved and no new errors are introduced.
Soft Skills for Full-Stack Developers: Full Stack Developer Career Path And Required Skills
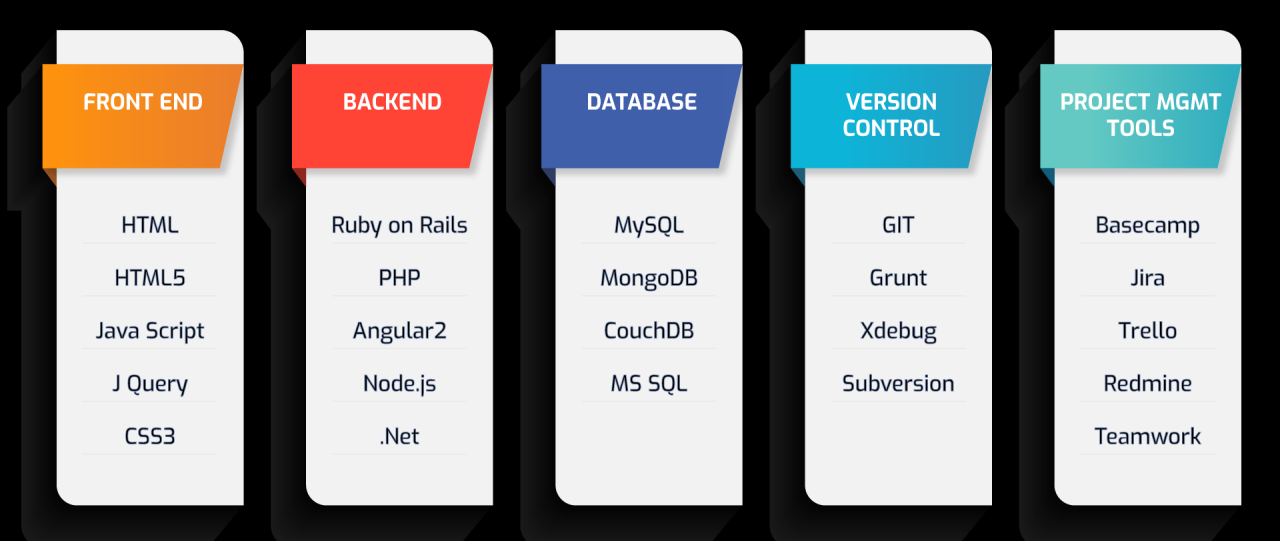
Technical skills are crucial for a full-stack developer, but soft skills are equally important for success. They determine how effectively you collaborate, communicate, and manage your work, ultimately impacting project outcomes and team dynamics. Mastering these skills elevates you from a competent coder to a valuable and sought-after team member.
Communication Skills in Development Teams
Effective communication is the cornerstone of any successful software development project. In a full-stack role, you’ll interact with designers, back-end developers, front-end developers, project managers, and clients. Clearly articulating technical concepts to non-technical stakeholders, actively listening to feedback, and providing constructive criticism are all vital for smooth collaboration and avoiding misunderstandings. For example, explaining a complex bug to a client requires simplifying technical jargon and focusing on the impact on the user experience.
Conversely, understanding a designer’s vision for a user interface requires attentive listening and clarifying questions to ensure accurate implementation.
Teamwork and Collaboration
Full-stack development rarely happens in isolation. You’ll be part of a team, working alongside others to achieve common goals. Collaboration involves sharing knowledge, providing support, and respecting diverse perspectives. Teamwork fosters a positive work environment, improves code quality through peer reviews, and accelerates project completion. For instance, pair programming allows developers to learn from each other, identify potential issues early, and create more robust code.
Effective collaboration tools, such as project management software and version control systems, further enhance team productivity.
Problem-Solving and Critical Thinking
Full-stack development is inherently problem-solving. You’ll constantly encounter unexpected challenges, bugs, and design limitations. Critical thinking allows you to analyze situations objectively, identify root causes, and develop effective solutions. This involves breaking down complex problems into smaller, manageable parts, evaluating different approaches, and choosing the most efficient and effective solution. A real-world example would be debugging a performance bottleneck in a web application, requiring the developer to analyze server logs, network traffic, and code to pinpoint the source of the issue and implement an appropriate fix.
Time Management and Organizational Skills
Full-stack developers juggle multiple tasks simultaneously – from front-end development to back-end programming, database management, and deployment. Effective time management and organizational skills are essential to prioritize tasks, meet deadlines, and avoid burnout. Utilizing project management tools, setting realistic goals, and breaking down large tasks into smaller, manageable steps are crucial for staying on track. For example, using a Kanban board to visualize tasks and track progress can significantly improve productivity and organization.
Similarly, adhering to agile methodologies promotes iterative development and efficient time management.
Examples of Soft Skills Contributing to Successful Project Completion
Successful project completion relies heavily on the effective application of soft skills. For example, a developer with strong communication skills can effectively convey project updates to stakeholders, preventing misunderstandings and ensuring alignment. A collaborative team that actively shares knowledge and supports each other will complete projects faster and with higher quality. Similarly, a developer with excellent problem-solving skills can efficiently resolve unexpected issues, minimizing project delays.
Finally, strong time management and organizational skills ensure tasks are completed on time and within budget, contributing to the overall project success. A project that successfully launched on time and under budget, due to effective teamwork, clear communication, and proactive problem-solving, serves as a testament to the importance of soft skills.
So you’re thinking about a full stack developer career path? It’s a great choice, requiring skills in both front-end and back-end development. To get a better idea of what you can earn and the overall job market, check out this resource on full stack developer salary expectations and job outlook. Knowing the salary potential and demand will help you plan your skill development and career progression more effectively.
Mastering languages like JavaScript, Python, and SQL is key to success in this rewarding field.
Outcome Summary
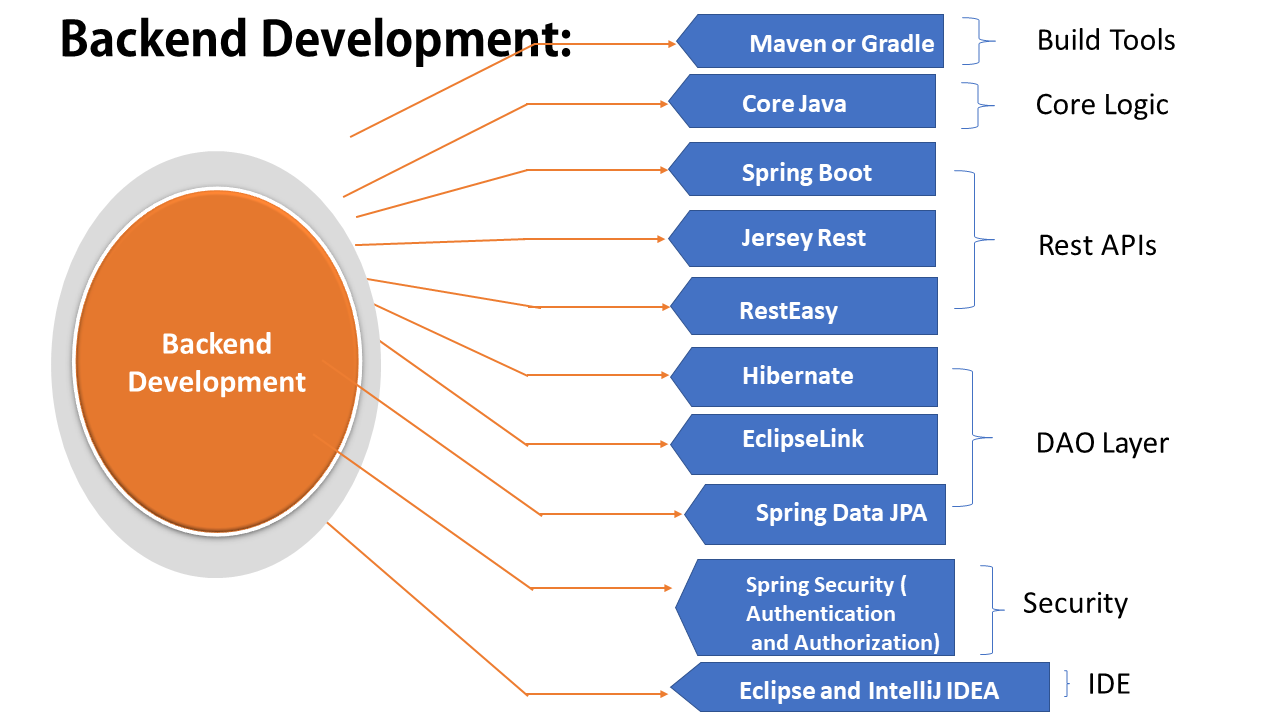
Mastering the full-stack developer career path is a challenging but incredibly rewarding endeavor. By diligently honing your skills in front-end, back-end, and DevOps technologies, along with cultivating essential soft skills, you can build a successful and fulfilling career. Remember, continuous learning is key in this ever-evolving field. Stay updated with the latest technologies and trends, and embrace the challenges that come with building complex and innovative applications.
The journey is demanding, but the potential rewards are immense.
FAQ Resource
What’s the average salary for a full-stack developer?
Salaries vary widely based on experience, location, and company size. Expect a range from entry-level to senior-level compensation.
How long does it take to become a full-stack developer?
The timeframe depends on your prior experience and learning pace. It can range from several months to several years of dedicated study and practice.
Which programming language should I learn first?
There’s no single “best” language. Popular choices include JavaScript, Python, and Java. Choose one based on your interests and career goals.
What are some good resources for learning full-stack development?
Online courses (Codecademy, Udemy, Coursera), bootcamps, and personal projects are all excellent resources. Experiment and find what works best for you.